Logomaker quick example
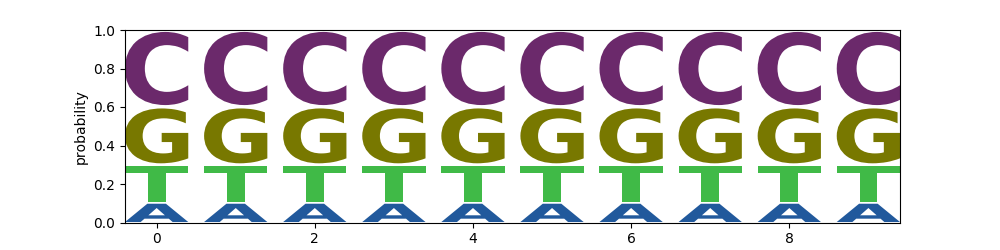
I wanted to use Logomaker to make a sequence logo. While the documentation is pretty good there wasn’t an example that showed exactly what I wanted. Most likely this is obvious if you’re familiar with Pandas.
The code below will load sequence probabilities from a tsv file, create a sequence logo and save it to logo.png
import numpy as np
import pandas as pd
import matplotlib.pyplot as plt
import logomaker
ss_df = pd.read_csv('all.tsv', sep="\t", index_col=0)
# create Logo object
ss_logo = logomaker.Logo(ss_df,
width=.8,
color_scheme='NajafabadiEtAl2017',
vpad=.05)
ss_logo.ax.set_ylabel('probability')
plt.savefig('logo.png')
all.tsv contains the following:
pos A T G C
0 0.1 0.2 0.3 0.4
1 0.1 0.2 0.3 0.4
2 0.1 0.2 0.3 0.4
3 0.1 0.2 0.3 0.4
4 0.1 0.2 0.3 0.4
5 0.1 0.2 0.3 0.4
6 0.1 0.2 0.3 0.4
7 0.1 0.2 0.3 0.4
8 0.1 0.2 0.3 0.4
9 0.1 0.2 0.3 0.4